리액트에 리덕스 툴킷 사용해 보기 타입스크립트 바이트
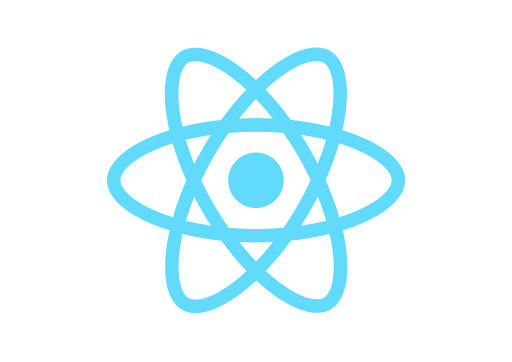
이번 글을 통해 배워갈 내용
- 리덕스 소개
- 리덕스 설치
- 샘플 리덕스 적용 코드
리덕스 소개
Redux는 Javascript 기반 앱에 상태 관리 라이브러리입니다.
앱의 데이터를 하나의 저장소(Store)에 관리해서
앱의 상태를 관리하는데 도움을 줍니다.
Redux Toolkit은 Redux의 보조 패키지로
Redux의 설정과 보일러 플레이트 코드를 최소화하는데 도움을 줍니다
리덕스 설치
필요시 아래와 같이 입력해서 샘플 프로젝트를 생성합니다
(npm 7+ 이상 버전에 최신버전 vite를 템플릿과 함께 react 타입스크립트로 생성)
npm create vite@latest kimc-sample -- --template react-ts
리덕스와 리덕스 툴킷을 설치합니다
(Redux 8.0.0 버전부터 타입스크립트로 제공이 돼서 @types/react-redux가 필요하지 않습니다)
npm i react-redux @reduxjs/toolkit
샘플 리덕스 적용 코드
index.ts
// index.ts
// Redux Toolkit에서 제공하는 configureStore 함수를 가져옵니다.
import { configureStore } from '@reduxjs/toolkit';
// 'sampleSlice'라는 Redux 리듀서를 가져옵니다. 이 리듀서는 나중에 스토어에 등록됩니다.
import sampleReducer from './sampleSlice';
// Redux 스토어를 설정하고 구성합니다.
export const store = configureStore({
reducer: {
// 'sampleReducer'를 'reducer' 객체에 등록합니다.
sampleReducer,
},
});
// 'store.dispatch'의 타입을 정의합니다. 이것은 Redux 스토어의 dispatch 메서드의 타입입니다.
export type AppDispatch = typeof store.dispatch;
// 'store.getState'의 반환 타입을 정의합니다. 이것은 Redux 스토어의 상태의 타입입니다.
export type RootState = ReturnType<typeof store.getState>;
hook.ts
// hook.ts
// React-Redux에서 제공하는 훅 및 타입을 가져옵니다.
import { TypedUseSelectorHook, useDispatch, useSelector } from 'react-redux';
// 이전에 정의한 'AppDispatch' 및 'RootState' 타입을 가져옵니다.
import { AppDispatch, RootState } from '.';
// 'useDispatch'를 이용하여 'AppDispatch' 타입을 가진 커스텀 'useAppDispatch' 훅을 정의합니다.
export const useAppDispatch = () => useDispatch<AppDispatch>();
// 'useSelector'를 사용하여 'RootState' 타입을 가진 커스텀 'useAppSelector' 훅을 정의합니다.
export const useAppSelector: TypedUseSelectorHook<RootState> = useSelector;
sampleSlice.ts
// sampleSlice.ts
// Redux Toolkit에서 제공하는 함수 및 타입을 가져옵니다.
import { createSlice, PayloadAction } from '@reduxjs/toolkit';
// 이전에 정의한 'RootState' 타입을 가져옵니다.
import { RootState } from '.';
// Redux 스토어에서 관리할 'SampleDto' 인터페이스를 정의합니다.
export interface SampleDto {
id: number;
name: string;
}
// 초기 상태를 정의합니다.
const initialState: SampleDto = {
id: 0,
name: 'not used',
};
// Redux Slice를 생성합니다.
export const sampleSlice = createSlice({
name: 'sample',
initialState,
reducers: {
// 'updateName' 액션을 정의합니다. 이 액션은 'name' 필드를 업데이트합니다.
updateName: (state, action: PayloadAction<string>) => {
state.name = action.payload;
},
},
});
// 생성한 액션을 가져옵니다.
export const { updateName } = sampleSlice.actions;
// Redux 스토어에서 'sampleReducer'의 상태를 선택하는 선택자를 정의합니다.
export const sampleSelector = (state: RootState) => state.sampleReducer;
// Redux Slice 리듀서를 내보냅니다.
export default sampleSlice.reducer;
Comp.tsx
// Comp.tsx
import { useAppSelector, useAppDispatch } from '../store/hook';
import { sampleSelector, updateName } from '../store/sampleSlice';
const SampleComponent = () => {
// Redux 스토어에서 'sampleSelector'를 사용하여 'selectedsample' 상태를 선택합니다.
const selectedsample = useAppSelector(sampleSelector);
// Redux 스토어에서 'useAppDispatch'를 사용하여 'dispatch' 함수를 가져옵니다.
const dispatch = useAppDispatch();
// 랜덤한 이름 목록을 정의합니다.
const names = ['Kimc', 'Bob', 'Gomc', 'Domc', 'Eve', 'Kamc', 'Grace'];
// 랜덤한 이름을 반환하는 함수를 정의합니다.
function getRandomName() {
const randomIndex = Math.floor(Math.random() * names.length);
return names[randomIndex];
}
// 'updateToRandomName' 함수를 정의합니다. 이 함수는 'updateName' 액션을 디스패치하여 'name' 필드를 랜덤한 이름으로 업데이트합니다.
const updateToRandomName = () => {
dispatch(updateName(getRandomName()));
};
return (
<button onClick={updateToRandomName} type="button">
{/* selectedsample 에 'name' 필드 값을 표시합니다. */}
{selectedsample.name}
</button>
);
};
main.tsx
// main.tsx
import React from 'react';
import ReactDOM from 'react-dom/client';
import App from './App.tsx';
import './index.css';
import { Provider } from 'react-redux';
import { store } from './store/index.ts';
// 'ReactDOM.createRoot'를 사용하여 React 애플리케이션의 루트 엘리먼트를 가져오고, 'render' 메서드로 애플리케이션을 렌더링합니다.
ReactDOM.createRoot(document.getElementById('root')!).render(
<React.StrictMode>
{/* Redux 스토어를 React 애플리케이션에 제공합니다. */}
<Provider store={store}>
{/* 'App' 컴포넌트를 렌더링합니다. */}
<App />
</Provider>
</React.StrictMode>,
);
참조 및 인용
Redux - A predictable state container for JavaScript apps. | Redux
A predictable state container for JavaScript apps.
redux.js.org
https://codemasterkimc.tistory.com/50
300년차 개발자의 좋은 코드 5계명 (Clean Code)
이번 글을 통해 배워갈 내용 좋은 코드(Clean Code)를 작성하기 위해 개발자로서 생각해볼 5가지 요소를 알아보겠습니다. 개요 좋은 코드란 무엇일까요? 저는 자원이 한정적인 컴퓨터 세상에서 좋
codemasterkimc.tistory.com
'Javascript > React' 카테고리의 다른 글
Vite 리액트 카카오맵 다음 주소 조회 API 연동 및 컴포넌트 개발(TS) (0) | 2024.04.01 |
---|---|
react-alert 대신 react-toastify 사용해서 알림 표시해보기 (0) | 2023.10.05 |
React에 카카오 지도 넣는 방법 (초간단) (Client Side Render) (0) | 2023.06.18 |
React로 이미지 업로드 및 필터 적용하고 출력해보기 (1) | 2022.11.19 |
TypeScript와 함께 useReducer를 사용하는 방법 (0) | 2022.07.16 |
리액트에 리덕스 툴킷 사용해 보기 타입스크립트 바이트
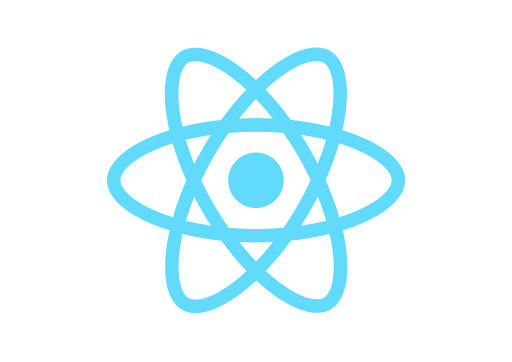
이번 글을 통해 배워갈 내용
- 리덕스 소개
- 리덕스 설치
- 샘플 리덕스 적용 코드
리덕스 소개
Redux는 Javascript 기반 앱에 상태 관리 라이브러리입니다.
앱의 데이터를 하나의 저장소(Store)에 관리해서
앱의 상태를 관리하는데 도움을 줍니다.
Redux Toolkit은 Redux의 보조 패키지로
Redux의 설정과 보일러 플레이트 코드를 최소화하는데 도움을 줍니다
리덕스 설치
필요시 아래와 같이 입력해서 샘플 프로젝트를 생성합니다
(npm 7+ 이상 버전에 최신버전 vite를 템플릿과 함께 react 타입스크립트로 생성)
npm create vite@latest kimc-sample -- --template react-ts
리덕스와 리덕스 툴킷을 설치합니다
(Redux 8.0.0 버전부터 타입스크립트로 제공이 돼서 @types/react-redux가 필요하지 않습니다)
npm i react-redux @reduxjs/toolkit
샘플 리덕스 적용 코드
index.ts
// index.ts
// Redux Toolkit에서 제공하는 configureStore 함수를 가져옵니다.
import { configureStore } from '@reduxjs/toolkit';
// 'sampleSlice'라는 Redux 리듀서를 가져옵니다. 이 리듀서는 나중에 스토어에 등록됩니다.
import sampleReducer from './sampleSlice';
// Redux 스토어를 설정하고 구성합니다.
export const store = configureStore({
reducer: {
// 'sampleReducer'를 'reducer' 객체에 등록합니다.
sampleReducer,
},
});
// 'store.dispatch'의 타입을 정의합니다. 이것은 Redux 스토어의 dispatch 메서드의 타입입니다.
export type AppDispatch = typeof store.dispatch;
// 'store.getState'의 반환 타입을 정의합니다. 이것은 Redux 스토어의 상태의 타입입니다.
export type RootState = ReturnType<typeof store.getState>;
hook.ts
// hook.ts
// React-Redux에서 제공하는 훅 및 타입을 가져옵니다.
import { TypedUseSelectorHook, useDispatch, useSelector } from 'react-redux';
// 이전에 정의한 'AppDispatch' 및 'RootState' 타입을 가져옵니다.
import { AppDispatch, RootState } from '.';
// 'useDispatch'를 이용하여 'AppDispatch' 타입을 가진 커스텀 'useAppDispatch' 훅을 정의합니다.
export const useAppDispatch = () => useDispatch<AppDispatch>();
// 'useSelector'를 사용하여 'RootState' 타입을 가진 커스텀 'useAppSelector' 훅을 정의합니다.
export const useAppSelector: TypedUseSelectorHook<RootState> = useSelector;
sampleSlice.ts
// sampleSlice.ts
// Redux Toolkit에서 제공하는 함수 및 타입을 가져옵니다.
import { createSlice, PayloadAction } from '@reduxjs/toolkit';
// 이전에 정의한 'RootState' 타입을 가져옵니다.
import { RootState } from '.';
// Redux 스토어에서 관리할 'SampleDto' 인터페이스를 정의합니다.
export interface SampleDto {
id: number;
name: string;
}
// 초기 상태를 정의합니다.
const initialState: SampleDto = {
id: 0,
name: 'not used',
};
// Redux Slice를 생성합니다.
export const sampleSlice = createSlice({
name: 'sample',
initialState,
reducers: {
// 'updateName' 액션을 정의합니다. 이 액션은 'name' 필드를 업데이트합니다.
updateName: (state, action: PayloadAction<string>) => {
state.name = action.payload;
},
},
});
// 생성한 액션을 가져옵니다.
export const { updateName } = sampleSlice.actions;
// Redux 스토어에서 'sampleReducer'의 상태를 선택하는 선택자를 정의합니다.
export const sampleSelector = (state: RootState) => state.sampleReducer;
// Redux Slice 리듀서를 내보냅니다.
export default sampleSlice.reducer;
Comp.tsx
// Comp.tsx
import { useAppSelector, useAppDispatch } from '../store/hook';
import { sampleSelector, updateName } from '../store/sampleSlice';
const SampleComponent = () => {
// Redux 스토어에서 'sampleSelector'를 사용하여 'selectedsample' 상태를 선택합니다.
const selectedsample = useAppSelector(sampleSelector);
// Redux 스토어에서 'useAppDispatch'를 사용하여 'dispatch' 함수를 가져옵니다.
const dispatch = useAppDispatch();
// 랜덤한 이름 목록을 정의합니다.
const names = ['Kimc', 'Bob', 'Gomc', 'Domc', 'Eve', 'Kamc', 'Grace'];
// 랜덤한 이름을 반환하는 함수를 정의합니다.
function getRandomName() {
const randomIndex = Math.floor(Math.random() * names.length);
return names[randomIndex];
}
// 'updateToRandomName' 함수를 정의합니다. 이 함수는 'updateName' 액션을 디스패치하여 'name' 필드를 랜덤한 이름으로 업데이트합니다.
const updateToRandomName = () => {
dispatch(updateName(getRandomName()));
};
return (
<button onClick={updateToRandomName} type="button">
{/* selectedsample 에 'name' 필드 값을 표시합니다. */}
{selectedsample.name}
</button>
);
};
main.tsx
// main.tsx
import React from 'react';
import ReactDOM from 'react-dom/client';
import App from './App.tsx';
import './index.css';
import { Provider } from 'react-redux';
import { store } from './store/index.ts';
// 'ReactDOM.createRoot'를 사용하여 React 애플리케이션의 루트 엘리먼트를 가져오고, 'render' 메서드로 애플리케이션을 렌더링합니다.
ReactDOM.createRoot(document.getElementById('root')!).render(
<React.StrictMode>
{/* Redux 스토어를 React 애플리케이션에 제공합니다. */}
<Provider store={store}>
{/* 'App' 컴포넌트를 렌더링합니다. */}
<App />
</Provider>
</React.StrictMode>,
);
참조 및 인용
Redux - A predictable state container for JavaScript apps. | Redux
A predictable state container for JavaScript apps.
redux.js.org
https://codemasterkimc.tistory.com/50
300년차 개발자의 좋은 코드 5계명 (Clean Code)
이번 글을 통해 배워갈 내용 좋은 코드(Clean Code)를 작성하기 위해 개발자로서 생각해볼 5가지 요소를 알아보겠습니다. 개요 좋은 코드란 무엇일까요? 저는 자원이 한정적인 컴퓨터 세상에서 좋
codemasterkimc.tistory.com
'Javascript > React' 카테고리의 다른 글
Vite 리액트 카카오맵 다음 주소 조회 API 연동 및 컴포넌트 개발(TS) (0) | 2024.04.01 |
---|---|
react-alert 대신 react-toastify 사용해서 알림 표시해보기 (0) | 2023.10.05 |
React에 카카오 지도 넣는 방법 (초간단) (Client Side Render) (0) | 2023.06.18 |
React로 이미지 업로드 및 필터 적용하고 출력해보기 (1) | 2022.11.19 |
TypeScript와 함께 useReducer를 사용하는 방법 (0) | 2022.07.16 |