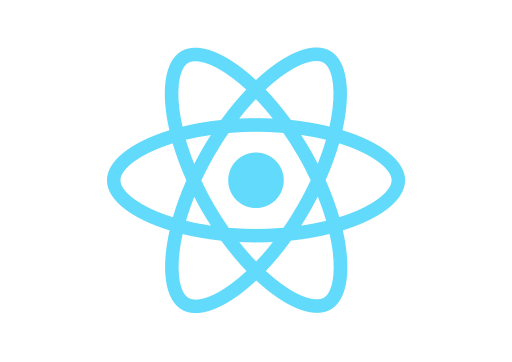
이번 글을 통해 배워갈 내용
- 리액트에 카카오 지도 넣는 방법 (vite)
1. 개발자 계정을 만들고
JavaScript 키를 발급받는다
Kakao Developers
카카오 API를 활용하여 다양한 어플리케이션을 개발해보세요. 카카오 로그인, 메시지 보내기, 친구 API, 인공지능 API 등을 제공합니다.
developers.kakao.com
2.
발급받은 Javascript 키를 index.html 파일에 넣는다.
SSR의 경우 dynamic import 등을 사용해서 해당되는 파일변경
<script type="text/javascript" src="//dapi.kakao.com/v2/maps/sdk.js?appkey=발급받은 APP KEY를 넣으시면 됩니다."></script>
3.
해당되는 컴포넌트 작성
필요시 커스텀 타입 정의
import { useEffect, useRef } from 'react';
const geoLocation = {
lat: 36.7228,
lng: 126.849613,
};
const markName = '샘플';
declare global {
interface Window {
kakao: any;
}
}
interface Marker {
name: string;
lat: number;
lng: number;
active: boolean;
point: number;
map: any;
}
export default function KakaoLocation() {
const mapRef = useRef<HTMLDivElement>(null);
useEffect(() => {
const initializeMap = () => {
const container = mapRef.current;
const { kakao } = window;
if (container && kakao) {
const options = {
center: new kakao.maps.LatLng(geoLocation.lat, geoLocation.lng),
level: 4,
};
const map = new kakao.maps.Map(container, options);
const marker: Marker = {
name: markName,
lat: geoLocation.lat,
lng: geoLocation.lng,
active: true,
point: 1,
map,
};
displayMarker(marker);
const position = new kakao.maps.LatLng(
geoLocation.lat,
geoLocation.lng
);
map.setCenter(position);
}
};
initializeMap();
}, []);
const displayMarker = (marker: Marker) => {
const { kakao } = window;
if (kakao) {
const iwPosition = new kakao.maps.LatLng(marker.lat, marker.lng);
const inactiveInfoWindow = `<div><span>${marker.name}</span></div>`;
new kakao.maps.InfoWindow({
zIndex: 1,
position: iwPosition,
content: inactiveInfoWindow,
disableAutoPan: false,
map: marker.map,
});
}
};
return (
<div
ref={mapRef}
style={{ width: '80%', height: '100vh', margin: '0 auto' }}
/>
);
}
4.
<KakaoLocation/> 컴포넌트 외부에서 import 및 사용
참조 및 인용
https://apis.map.kakao.com/web/guide/
'Javascript > React' 카테고리의 다른 글
react-alert 대신 react-toastify 사용해서 알림 표시해보기 (0) | 2023.10.05 |
---|---|
React에 Redux Toolkit 사용해보기 Typescript Vite (0) | 2023.10.05 |
React로 이미지 업로드 및 필터 적용하고 출력해보기 (1) | 2022.11.19 |
TypeScript와 함께 useReducer를 사용하는 방법 (0) | 2022.07.16 |
리액트에서 여러 체크박스들을 하나의 컴포넌트로 재활용 해보기 ts (0) | 2022.06.22 |
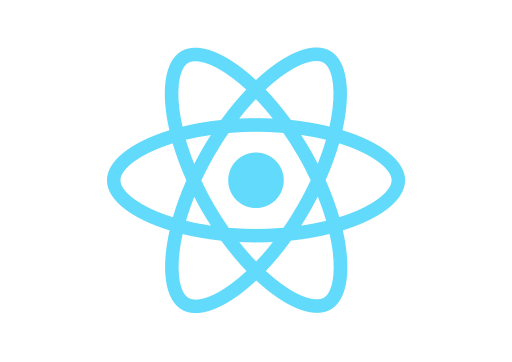
이번 글을 통해 배워갈 내용
- 리액트에 카카오 지도 넣는 방법 (vite)
1. 개발자 계정을 만들고
JavaScript 키를 발급받는다
Kakao Developers
카카오 API를 활용하여 다양한 어플리케이션을 개발해보세요. 카카오 로그인, 메시지 보내기, 친구 API, 인공지능 API 등을 제공합니다.
developers.kakao.com
2.
발급받은 Javascript 키를 index.html 파일에 넣는다.
SSR의 경우 dynamic import 등을 사용해서 해당되는 파일변경
<script type="text/javascript" src="//dapi.kakao.com/v2/maps/sdk.js?appkey=발급받은 APP KEY를 넣으시면 됩니다."></script>
3.
해당되는 컴포넌트 작성
필요시 커스텀 타입 정의
import { useEffect, useRef } from 'react';
const geoLocation = {
lat: 36.7228,
lng: 126.849613,
};
const markName = '샘플';
declare global {
interface Window {
kakao: any;
}
}
interface Marker {
name: string;
lat: number;
lng: number;
active: boolean;
point: number;
map: any;
}
export default function KakaoLocation() {
const mapRef = useRef<HTMLDivElement>(null);
useEffect(() => {
const initializeMap = () => {
const container = mapRef.current;
const { kakao } = window;
if (container && kakao) {
const options = {
center: new kakao.maps.LatLng(geoLocation.lat, geoLocation.lng),
level: 4,
};
const map = new kakao.maps.Map(container, options);
const marker: Marker = {
name: markName,
lat: geoLocation.lat,
lng: geoLocation.lng,
active: true,
point: 1,
map,
};
displayMarker(marker);
const position = new kakao.maps.LatLng(
geoLocation.lat,
geoLocation.lng
);
map.setCenter(position);
}
};
initializeMap();
}, []);
const displayMarker = (marker: Marker) => {
const { kakao } = window;
if (kakao) {
const iwPosition = new kakao.maps.LatLng(marker.lat, marker.lng);
const inactiveInfoWindow = `<div><span>${marker.name}</span></div>`;
new kakao.maps.InfoWindow({
zIndex: 1,
position: iwPosition,
content: inactiveInfoWindow,
disableAutoPan: false,
map: marker.map,
});
}
};
return (
<div
ref={mapRef}
style={{ width: '80%', height: '100vh', margin: '0 auto' }}
/>
);
}
4.
<KakaoLocation/> 컴포넌트 외부에서 import 및 사용
참조 및 인용
https://apis.map.kakao.com/web/guide/
'Javascript > React' 카테고리의 다른 글
react-alert 대신 react-toastify 사용해서 알림 표시해보기 (0) | 2023.10.05 |
---|---|
React에 Redux Toolkit 사용해보기 Typescript Vite (0) | 2023.10.05 |
React로 이미지 업로드 및 필터 적용하고 출력해보기 (1) | 2022.11.19 |
TypeScript와 함께 useReducer를 사용하는 방법 (0) | 2022.07.16 |
리액트에서 여러 체크박스들을 하나의 컴포넌트로 재활용 해보기 ts (0) | 2022.06.22 |