```
자바 고전적 For loop 대신 IntStream 사용해보기
```
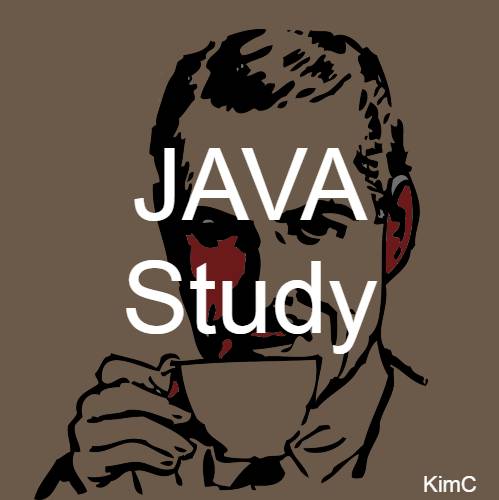
이번 글을 통해 배워갈 내용
- IntStream 사용해보기
Stream 이란
Stream에 대한 설명은 아래 문서를 참조해주시면 됩니다.
https://codemasterkimc.tistory.com/391
For loop 대신 Stream을 사용하는 2가지 이유
``` For loop 대신 Stream을 사용하는 2가지 이유 ``` 이번 글을 통해 배워갈 내용 Stream에 대한 설명 For loop와 비교 Stream을 사용하는 두 가지 이유 Stream에 대한 설명 자바에서는 데이터를 처..
codemasterkimc.tistory.com
IntStream 사용해보기
of를 사용해서 특정 숫자들을 순차적으로 사용할 수도 있고
import java.util.stream.IntStream;
public class App {
public static void main(String[] args) {
IntStream.of(1, 3, 5, 7).forEach(System.out::print); // 1357 출력
}
}
range를 사용해서 마지막 수를 포함하지 않는 범위를 출력하거나
rangeClosed를 사용해서 마지막 수를 포함하는 범위를 출력할 수 있습니다.
import java.util.stream.IntStream;
public class App {
public static void main(String[] args) {
IntStream.range(0, 5).forEach(System.out::print); // 01234 출력
System.out.println();
IntStream.rangeClosed(0, 5).forEach(System.out::print); // 012345 출력
}
}
iterator를 이용해서 이전 값의 영향을 받는 Stream을 만들고
limit을 써서 개수 제한을 할수 있습니다.
import java.util.stream.IntStream;
public class App {
public static void main(String[] args) {
IntStream.iterate(1, i->i*2).limit(5).forEach(System.out::print); // 124816 출력
}
}
혹은
generate 를 써서 이전 값의 영향을 안 받아도 되는 방법의 Stream 도 가능합니다.
import java.util.concurrent.ThreadLocalRandom;
import java.util.stream.IntStream;
public class App {
public static void main(String[] args) {
IntStream.generate(()-> ThreadLocalRandom.current().nextInt(5)).limit(5).forEach(System.out::print);
}
}
또한
map()
mapToObject()
boxed()
mapToDouble()
mapToLong()
anyMatch()
allMatch()
noneMatch()
filter()
reduce()
등이 있으며
예시로 5의 팩토리얼을 구하고자 한다면
import java.util.stream.IntStream;
public class App {
public static void main(String[] args) {
System.out.print(IntStream.rangeClosed(1, 5).reduce(1, (x, y) -> x * y));
}
}
위와 같이 쓸수 있습니다.
읽어주셔서 감사합니다
무엇인가 얻어가셨기를 바라며
오늘도 즐거운 코딩 하시길 바랍니다 ~ :)
참조
https://stackoverflow.com/questions/23396033/random-over-threadlocalrandom
Random over ThreadLocalRandom
Instances of java.util.Random are threadsafe. However, the concurrent use of the same java.util.Random instance across threads may encounter contention and consequent poor performance. Consider
stackoverflow.com
https://docs.oracle.com/javase/8/docs/api/java/util/stream/IntStream.html
IntStream (Java Platform SE 8 )
Returns an infinite sequential ordered IntStream produced by iterative application of a function f to an initial element seed, producing a Stream consisting of seed, f(seed), f(f(seed)), etc. The first element (position 0) in the IntStream will be the prov
docs.oracle.com
https://www.geeksforgeeks.org/intstream-of-in-java/
IntStream of() in Java - GeeksforGeeks
A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
www.geeksforgeeks.org
https://www.baeldung.com/java-intstream-convert
Java IntStream Conversions | Baeldung
Learn how to work with integer streams using the Java Stream API.
www.baeldung.com
'Java > Java 기타' 카테고리의 다른 글
자바에서 @Override 사용하는 한 가지 방법 (0) | 2022.05.21 |
---|---|
JAVA Reducer로 소문자 열 대문자 변환하며 더하는 2가지 방법 (0) | 2022.05.18 |
For loop 대신 Stream을 사용하는 2가지 이유 (0) | 2022.05.15 |
JAVA 공백으로 띄운 스트링 입력 정수 리스트로 변환하는 3가지 방법 (0) | 2022.05.07 |
StringBuffer를 쓰는 두가지 이유 그리고 간략한 JAVA StringBuilder, StringBuffer, String 설명 (0) | 2022.03.26 |
```
자바 고전적 For loop 대신 IntStream 사용해보기
```
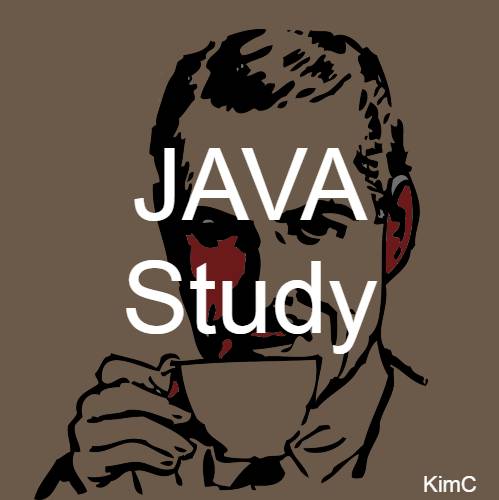
이번 글을 통해 배워갈 내용
- IntStream 사용해보기
Stream 이란
Stream에 대한 설명은 아래 문서를 참조해주시면 됩니다.
https://codemasterkimc.tistory.com/391
For loop 대신 Stream을 사용하는 2가지 이유
``` For loop 대신 Stream을 사용하는 2가지 이유 ``` 이번 글을 통해 배워갈 내용 Stream에 대한 설명 For loop와 비교 Stream을 사용하는 두 가지 이유 Stream에 대한 설명 자바에서는 데이터를 처..
codemasterkimc.tistory.com
IntStream 사용해보기
of를 사용해서 특정 숫자들을 순차적으로 사용할 수도 있고
import java.util.stream.IntStream;
public class App {
public static void main(String[] args) {
IntStream.of(1, 3, 5, 7).forEach(System.out::print); // 1357 출력
}
}
range를 사용해서 마지막 수를 포함하지 않는 범위를 출력하거나
rangeClosed를 사용해서 마지막 수를 포함하는 범위를 출력할 수 있습니다.
import java.util.stream.IntStream;
public class App {
public static void main(String[] args) {
IntStream.range(0, 5).forEach(System.out::print); // 01234 출력
System.out.println();
IntStream.rangeClosed(0, 5).forEach(System.out::print); // 012345 출력
}
}
iterator를 이용해서 이전 값의 영향을 받는 Stream을 만들고
limit을 써서 개수 제한을 할수 있습니다.
import java.util.stream.IntStream;
public class App {
public static void main(String[] args) {
IntStream.iterate(1, i->i*2).limit(5).forEach(System.out::print); // 124816 출력
}
}
혹은
generate 를 써서 이전 값의 영향을 안 받아도 되는 방법의 Stream 도 가능합니다.
import java.util.concurrent.ThreadLocalRandom;
import java.util.stream.IntStream;
public class App {
public static void main(String[] args) {
IntStream.generate(()-> ThreadLocalRandom.current().nextInt(5)).limit(5).forEach(System.out::print);
}
}
또한
map()
mapToObject()
boxed()
mapToDouble()
mapToLong()
anyMatch()
allMatch()
noneMatch()
filter()
reduce()
등이 있으며
예시로 5의 팩토리얼을 구하고자 한다면
import java.util.stream.IntStream;
public class App {
public static void main(String[] args) {
System.out.print(IntStream.rangeClosed(1, 5).reduce(1, (x, y) -> x * y));
}
}
위와 같이 쓸수 있습니다.
읽어주셔서 감사합니다
무엇인가 얻어가셨기를 바라며
오늘도 즐거운 코딩 하시길 바랍니다 ~ :)
참조
https://stackoverflow.com/questions/23396033/random-over-threadlocalrandom
Random over ThreadLocalRandom
Instances of java.util.Random are threadsafe. However, the concurrent use of the same java.util.Random instance across threads may encounter contention and consequent poor performance. Consider
stackoverflow.com
https://docs.oracle.com/javase/8/docs/api/java/util/stream/IntStream.html
IntStream (Java Platform SE 8 )
Returns an infinite sequential ordered IntStream produced by iterative application of a function f to an initial element seed, producing a Stream consisting of seed, f(seed), f(f(seed)), etc. The first element (position 0) in the IntStream will be the prov
docs.oracle.com
https://www.geeksforgeeks.org/intstream-of-in-java/
IntStream of() in Java - GeeksforGeeks
A Computer Science portal for geeks. It contains well written, well thought and well explained computer science and programming articles, quizzes and practice/competitive programming/company interview Questions.
www.geeksforgeeks.org
https://www.baeldung.com/java-intstream-convert
Java IntStream Conversions | Baeldung
Learn how to work with integer streams using the Java Stream API.
www.baeldung.com
'Java > Java 기타' 카테고리의 다른 글
자바에서 @Override 사용하는 한 가지 방법 (0) | 2022.05.21 |
---|---|
JAVA Reducer로 소문자 열 대문자 변환하며 더하는 2가지 방법 (0) | 2022.05.18 |
For loop 대신 Stream을 사용하는 2가지 이유 (0) | 2022.05.15 |
JAVA 공백으로 띄운 스트링 입력 정수 리스트로 변환하는 3가지 방법 (0) | 2022.05.07 |
StringBuffer를 쓰는 두가지 이유 그리고 간략한 JAVA StringBuilder, StringBuffer, String 설명 (0) | 2022.03.26 |