```
백준 12607번, 12608번 T9 Spelling C++ 구현해보기
```
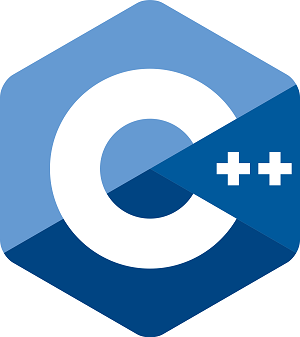
이번 글을 통해 배워갈 내용
- 백준 12607번 T9 Spelling (Small) C++ 풀이
- 백준 12608번 T9 Spelling (Large) C++ 풀이
https://www.acmicpc.net/problem/12607
12607번: T9 Spelling (Small)
The Latin alphabet contains 26 characters and telephones only have ten digits on the keypad. We would like to make it easier to write a message to your friend using a sequence of keypresses to indicate the desired characters. The letters are mapped onto th
www.acmicpc.net
https://www.acmicpc.net/problem/12608
12608번: T9 Spelling (Large)
The Latin alphabet contains 26 characters and telephones only have ten digits on the keypad. We would like to make it easier to write a message to your friend using a sequence of keypresses to indicate the desired characters. The letters are mapped onto th
www.acmicpc.net
백준 12607번 T9 Spelling (Small)과 (Large)는
난이도 브론즈 등급의 문제로서
테스트 케이스만큼 알파벳 소문자와 공란이 주어질 때
이를 숫자로 변환해주어야 하는 문제입니다.
예를 들어
a는 2
b는 22
c 는 222
d는 3
...
z는 9999
입니다.
공란은 0으로 변환하며
앞에 지금 입력받는 숫자와 동일한 숫자가 있으면
한 칸 띄어줍니다.
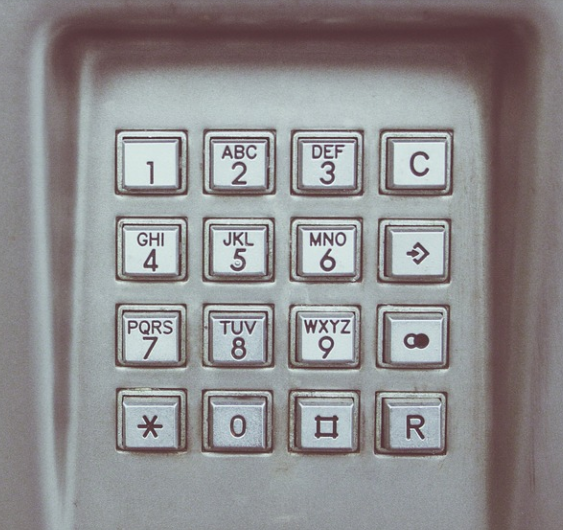
30분 정도 위에 링크를 방문하셔서 풀어보시고
안 풀리시는 경우에만 아래 해답을 봐주시면 감사하겠습니다.
입력받고 입력받은 데이터를 스위치 케이스로 가공해서 출력하면 되는 쉬운 문제였습니다.
풀이를 더 효율적으로 하면 a 부터 z까지 변환하는 데이터를 함수와 분리해서 배열로 넣으면 더 좋겠지만
시간 관계상 함수와 붙여서 풀었습니다.
전체 코드는 다음과 같습니다(두 문제 모두 답이 같음)
#include <iostream>
#include <string>
#include <sstream>
#include <vector>
#include <array>
#include <stack>
#include <queue>
#include <map>
#include <algorithm>
#include <numeric>
#include <cmath>
#include <regex>
namespace kimc::lib {
std::string find_sequence_of_keypresses(std::string inputStr)
{
std::string outputStr = " ";
for (char letter : inputStr)
{
std::string curStr = "";
switch (letter)
{
case 'a':
{
if (outputStr.back() == '2')
{
curStr += " ";
}
curStr += "2";
break;
}
case 'b':
{
if (outputStr.back() == '2')
{
curStr += " ";
}
curStr += "22";
break;
}
case 'c':
{
if (outputStr.back() == '2')
{
curStr += " ";
}
curStr += "222";
break;
}
case 'd':
{
if (outputStr.back() == '3')
{
curStr += " ";
}
curStr += "3";
break;
}
case 'e':
{
if (outputStr.back() == '3')
{
curStr += " ";
}
curStr += "33";
break;
}
case 'f':
{
if (outputStr.back() == '3')
{
curStr += " ";
}
curStr += "333";
break;
}
case 'g':
{
if (outputStr.back() == '4')
{
curStr += " ";
}
curStr += "4";
break;
}
case 'h':
{
if (outputStr.back() == '4')
{
curStr += " ";
}
curStr += "44";
break;
}
case 'i':
{
if (outputStr.back() == '4')
{
curStr += " ";
}
curStr += "444";
break;
}
case 'j':
{
if (outputStr.back() == '5')
{
curStr += " ";
}
curStr += "5";
break;
}
case 'k':
{
if (outputStr.back() == '5')
{
curStr += " ";
}
curStr += "55";
break;
}
case 'l':
{
if (outputStr.back() == '5')
{
curStr += " ";
}
curStr += "555";
break;
}
case 'm':
{
if (outputStr.back() == '6')
{
curStr += " ";
}
curStr += "6";
break;
}
case 'n':
{
if (outputStr.back() == '6')
{
curStr += " ";
}
curStr += "66";
break;
}
case 'o':
{
if (outputStr.back() == '6')
{
curStr += " ";
}
curStr += "666";
break;
}
case 'p':
{
if (outputStr.back() == '7')
{
curStr += " ";
}
curStr += "7";
break;
}
case 'q':
{
if (outputStr.back() == '7')
{
curStr += " ";
}
curStr += "77";
break;
}
case 'r':
{
if (outputStr.back() == '7')
{
curStr += " ";
}
curStr += "777";
break;
}
case 's':
{
if (outputStr.back() == '7')
{
curStr += " ";
}
curStr += "7777";
break;
}
case 't':
{
if (outputStr.back() == '8')
{
curStr += " ";
}
curStr += "8";
break;
}
case 'u':
{
if (outputStr.back() == '8')
{
curStr += " ";
}
curStr += "88";
break;
}
case 'v':
{
if (outputStr.back() == '8')
{
curStr += " ";
}
curStr += "888";
break;
}
case 'w':
{
if (outputStr.back() == '9')
{
curStr += " ";
}
curStr += "9";
break;
}
case 'x':
{
if (outputStr.back() == '9')
{
curStr += " ";
}
curStr += "99";
break;
}
case 'y':
{
if (outputStr.back() == '9')
{
curStr += " ";
}
curStr += "999";
break;
}
case 'z':
{
if (outputStr.back() == '9')
{
curStr += " ";
}
curStr += "9999";
break;
}
case ' ':
{
if (outputStr.back() == '0')
{
curStr += " ";
}
curStr += "0";
break;
}
default:
{
std::cout << "error";
break;
}
}
outputStr += curStr;
}
return outputStr;
}
}
int main()
{
// 입력 최적화
std::cin.tie(NULL);
std::ios::sync_with_stdio(false);
std::string inputStr;
std::getline(std::cin, inputStr);
std::stringstream ss(inputStr);
int32_t caseCount;
ss >> caseCount;
ss.clear();
ss.seekg(0);
for (int32_t i = 0; i < caseCount; i++)
{
std::getline(std::cin, inputStr);
ss.str(inputStr);
ss.clear();
ss.seekg(0);
std::string outputStr = "";
outputStr += "Case #" + std::to_string(i+1) + ":";
outputStr += kimc::lib::find_sequence_of_keypresses(inputStr);
outputStr += "\n";
std::cout << outputStr;
}
}
읽어주셔서 감사합니다
무엇인가 얻어가셨기를 바라며
오늘도 즐거운 코딩 하시길 바랍니다 ~ :)
참조 및 인용
C++ Primer
Introduction to Algorithms
https://codemasterkimc.tistory.com/35
C++ 이론을 배울수 있는 곳 정리
개요 C++을 배우는 책, 강의, 블로그, 링크 등을 공유합니다. (링크 및 간략한 설명을 하였으나 만약 원작자가 링크를 거는것을 원치 않을 경우 연락주시기 바랍니다.) 서적 https://www.amazon.com/Prime
codemasterkimc.tistory.com
https://codemasterkimc.tistory.com/50
300년차 개발자의 좋은 코드 5계명 (Clean Code)
이번 글을 통해 배워갈 내용 좋은 코드(Clean Code)를 작성하기 위해 개발자로서 생각해볼 5가지 요소를 알아보겠습니다. 개요 좋은 코드란 무엇일까요? 저는 자원이 한정적인 컴퓨터 세상에서 좋
codemasterkimc.tistory.com
'C++ > C++ 알고리즘' 카테고리의 다른 글
백준 12595번, 백준 12596번 Odd Man Out C++ 구현해보기 (0) | 2021.11.01 |
---|---|
백준 12603번, 백준 12604번 Store Credit C++ 구현해보기 (0) | 2021.10.31 |
백준 12605번, 12606번 단어순서 뒤집기 C++ 구현해보기 (0) | 2021.10.31 |
백준 17608번 막대기 C++ 구현해보기 (0) | 2021.10.31 |
백준 2525번 오븐시계 C++ 구현해보기 (0) | 2021.10.31 |
```
백준 12607번, 12608번 T9 Spelling C++ 구현해보기
```
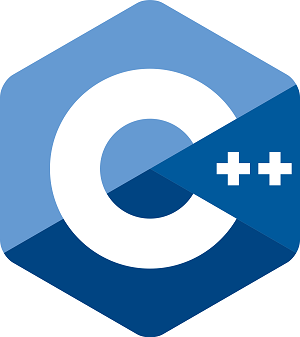
이번 글을 통해 배워갈 내용
- 백준 12607번 T9 Spelling (Small) C++ 풀이
- 백준 12608번 T9 Spelling (Large) C++ 풀이
https://www.acmicpc.net/problem/12607
12607번: T9 Spelling (Small)
The Latin alphabet contains 26 characters and telephones only have ten digits on the keypad. We would like to make it easier to write a message to your friend using a sequence of keypresses to indicate the desired characters. The letters are mapped onto th
www.acmicpc.net
https://www.acmicpc.net/problem/12608
12608번: T9 Spelling (Large)
The Latin alphabet contains 26 characters and telephones only have ten digits on the keypad. We would like to make it easier to write a message to your friend using a sequence of keypresses to indicate the desired characters. The letters are mapped onto th
www.acmicpc.net
백준 12607번 T9 Spelling (Small)과 (Large)는
난이도 브론즈 등급의 문제로서
테스트 케이스만큼 알파벳 소문자와 공란이 주어질 때
이를 숫자로 변환해주어야 하는 문제입니다.
예를 들어
a는 2
b는 22
c 는 222
d는 3
...
z는 9999
입니다.
공란은 0으로 변환하며
앞에 지금 입력받는 숫자와 동일한 숫자가 있으면
한 칸 띄어줍니다.
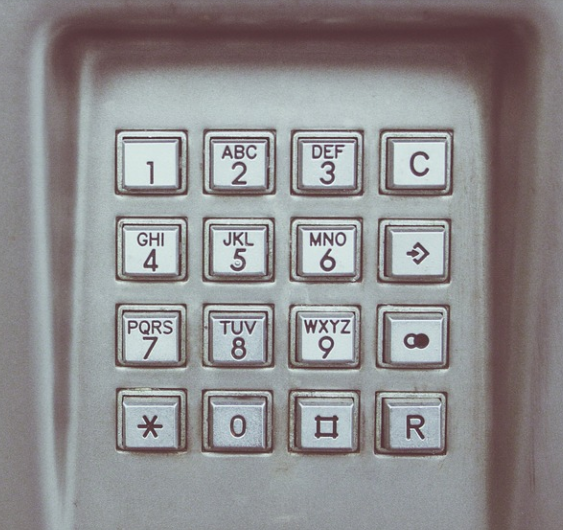
30분 정도 위에 링크를 방문하셔서 풀어보시고
안 풀리시는 경우에만 아래 해답을 봐주시면 감사하겠습니다.
입력받고 입력받은 데이터를 스위치 케이스로 가공해서 출력하면 되는 쉬운 문제였습니다.
풀이를 더 효율적으로 하면 a 부터 z까지 변환하는 데이터를 함수와 분리해서 배열로 넣으면 더 좋겠지만
시간 관계상 함수와 붙여서 풀었습니다.
전체 코드는 다음과 같습니다(두 문제 모두 답이 같음)
#include <iostream>
#include <string>
#include <sstream>
#include <vector>
#include <array>
#include <stack>
#include <queue>
#include <map>
#include <algorithm>
#include <numeric>
#include <cmath>
#include <regex>
namespace kimc::lib {
std::string find_sequence_of_keypresses(std::string inputStr)
{
std::string outputStr = " ";
for (char letter : inputStr)
{
std::string curStr = "";
switch (letter)
{
case 'a':
{
if (outputStr.back() == '2')
{
curStr += " ";
}
curStr += "2";
break;
}
case 'b':
{
if (outputStr.back() == '2')
{
curStr += " ";
}
curStr += "22";
break;
}
case 'c':
{
if (outputStr.back() == '2')
{
curStr += " ";
}
curStr += "222";
break;
}
case 'd':
{
if (outputStr.back() == '3')
{
curStr += " ";
}
curStr += "3";
break;
}
case 'e':
{
if (outputStr.back() == '3')
{
curStr += " ";
}
curStr += "33";
break;
}
case 'f':
{
if (outputStr.back() == '3')
{
curStr += " ";
}
curStr += "333";
break;
}
case 'g':
{
if (outputStr.back() == '4')
{
curStr += " ";
}
curStr += "4";
break;
}
case 'h':
{
if (outputStr.back() == '4')
{
curStr += " ";
}
curStr += "44";
break;
}
case 'i':
{
if (outputStr.back() == '4')
{
curStr += " ";
}
curStr += "444";
break;
}
case 'j':
{
if (outputStr.back() == '5')
{
curStr += " ";
}
curStr += "5";
break;
}
case 'k':
{
if (outputStr.back() == '5')
{
curStr += " ";
}
curStr += "55";
break;
}
case 'l':
{
if (outputStr.back() == '5')
{
curStr += " ";
}
curStr += "555";
break;
}
case 'm':
{
if (outputStr.back() == '6')
{
curStr += " ";
}
curStr += "6";
break;
}
case 'n':
{
if (outputStr.back() == '6')
{
curStr += " ";
}
curStr += "66";
break;
}
case 'o':
{
if (outputStr.back() == '6')
{
curStr += " ";
}
curStr += "666";
break;
}
case 'p':
{
if (outputStr.back() == '7')
{
curStr += " ";
}
curStr += "7";
break;
}
case 'q':
{
if (outputStr.back() == '7')
{
curStr += " ";
}
curStr += "77";
break;
}
case 'r':
{
if (outputStr.back() == '7')
{
curStr += " ";
}
curStr += "777";
break;
}
case 's':
{
if (outputStr.back() == '7')
{
curStr += " ";
}
curStr += "7777";
break;
}
case 't':
{
if (outputStr.back() == '8')
{
curStr += " ";
}
curStr += "8";
break;
}
case 'u':
{
if (outputStr.back() == '8')
{
curStr += " ";
}
curStr += "88";
break;
}
case 'v':
{
if (outputStr.back() == '8')
{
curStr += " ";
}
curStr += "888";
break;
}
case 'w':
{
if (outputStr.back() == '9')
{
curStr += " ";
}
curStr += "9";
break;
}
case 'x':
{
if (outputStr.back() == '9')
{
curStr += " ";
}
curStr += "99";
break;
}
case 'y':
{
if (outputStr.back() == '9')
{
curStr += " ";
}
curStr += "999";
break;
}
case 'z':
{
if (outputStr.back() == '9')
{
curStr += " ";
}
curStr += "9999";
break;
}
case ' ':
{
if (outputStr.back() == '0')
{
curStr += " ";
}
curStr += "0";
break;
}
default:
{
std::cout << "error";
break;
}
}
outputStr += curStr;
}
return outputStr;
}
}
int main()
{
// 입력 최적화
std::cin.tie(NULL);
std::ios::sync_with_stdio(false);
std::string inputStr;
std::getline(std::cin, inputStr);
std::stringstream ss(inputStr);
int32_t caseCount;
ss >> caseCount;
ss.clear();
ss.seekg(0);
for (int32_t i = 0; i < caseCount; i++)
{
std::getline(std::cin, inputStr);
ss.str(inputStr);
ss.clear();
ss.seekg(0);
std::string outputStr = "";
outputStr += "Case #" + std::to_string(i+1) + ":";
outputStr += kimc::lib::find_sequence_of_keypresses(inputStr);
outputStr += "\n";
std::cout << outputStr;
}
}
읽어주셔서 감사합니다
무엇인가 얻어가셨기를 바라며
오늘도 즐거운 코딩 하시길 바랍니다 ~ :)
참조 및 인용
C++ Primer
Introduction to Algorithms
https://codemasterkimc.tistory.com/35
C++ 이론을 배울수 있는 곳 정리
개요 C++을 배우는 책, 강의, 블로그, 링크 등을 공유합니다. (링크 및 간략한 설명을 하였으나 만약 원작자가 링크를 거는것을 원치 않을 경우 연락주시기 바랍니다.) 서적 https://www.amazon.com/Prime
codemasterkimc.tistory.com
https://codemasterkimc.tistory.com/50
300년차 개발자의 좋은 코드 5계명 (Clean Code)
이번 글을 통해 배워갈 내용 좋은 코드(Clean Code)를 작성하기 위해 개발자로서 생각해볼 5가지 요소를 알아보겠습니다. 개요 좋은 코드란 무엇일까요? 저는 자원이 한정적인 컴퓨터 세상에서 좋
codemasterkimc.tistory.com
'C++ > C++ 알고리즘' 카테고리의 다른 글
백준 12595번, 백준 12596번 Odd Man Out C++ 구현해보기 (0) | 2021.11.01 |
---|---|
백준 12603번, 백준 12604번 Store Credit C++ 구현해보기 (0) | 2021.10.31 |
백준 12605번, 12606번 단어순서 뒤집기 C++ 구현해보기 (0) | 2021.10.31 |
백준 17608번 막대기 C++ 구현해보기 (0) | 2021.10.31 |
백준 2525번 오븐시계 C++ 구현해보기 (0) | 2021.10.31 |