```
백준 2108번 통계학 C++ 구현해보기
```
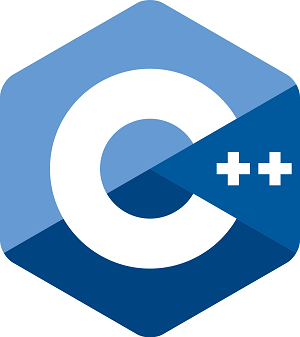
이번 글을 통해 배워갈 내용
- 백준 2108번 풀이
https://www.acmicpc.net/problem/2108
2108번: 통계학
첫째 줄에 수의 개수 N(1 ≤ N ≤ 500,000)이 주어진다. 단, N은 홀수이다. 그 다음 N개의 줄에는 정수들이 주어진다. 입력되는 정수의 절댓값은 4,000을 넘지 않는다.
www.acmicpc.net
백준 2108번 통계학은
난이도 실버 등급의 문제로서
설명
산술평균 Average(Mean),
중앙값 Median
최빈값 most frequent value
범위 range
를 구해주면 되는 문제입니다.
30분 정도 위에 링크를 방문하셔서 풀어보시고
안 풀리시는 경우에만 아래 해답을 봐주시면 감사하겠습니다.
산술평균은 간단하게 N개의 수의 합을 N으로 나눠주면 됩니다.
int32_t find_mean(const std::vector<int32_t> &ElemVec) {
int32_t sum = 0;
const size_t vecSize = ElemVec.size();
for (size_t i = 0; i < vecSize; i++)
{
sum += ElemVec[i];
}
const int32_t avg = floor((sum / static_cast<double_t>(vecSize)) + 0.5);
return avg;
}
중앙값은 데이터를 오름차순으로 정렬 후 데이터의 개수가 홀수면 중앙값, 짝수면 중앙에 있는 두 수의 합 나누기 2를 해주면 됩니다.(정렬된 데이터를 넣는다고 가정하고 함수를 작성했고 입력하는 곳에서 sort를 사용해 정렬했습니다)
int32_t find_median(const std::vector<int32_t>& ElemVec) {
const size_t vecSize = ElemVec.size();
int32_t retVal = 0;
if ((vecSize % 2) == 0)
{
retVal = floor(((ElemVec[vecSize / 2 - 1] + ElemVec[vecSize / 2]) / 2) + 0.5);
}
else
{
retVal = ElemVec[vecSize / 2];
}
return retVal;
}
최빈값은 가장 많이 나타나는 숫자를 구해주면 되는데 해당 문제의 경우 2개 이상일 경우 가장 많이 나타나는 수가 2개 이상일 경우 오름차순에서 두 번째 수를 반환해주면 됩니다.
int32_t find_most_frequent_value(const std::vector<int32_t>& ElemVec) {
std::map<int32_t, int32_t> counterMap;
for (auto it : ElemVec)
{
++counterMap[it];
}
int32_t maxVal = 0;
std::vector<int32_t> maxValVec;
for (auto it : counterMap)
{
if (it.second >= maxVal)
{
if (it.second != maxVal)
{
maxVal = it.second;
maxValVec.clear();
}
maxValVec.push_back(it.first);
}
}
int32_t retVal = maxValVec[0];
if (maxValVec.size() >= 2)
{
retVal = maxValVec[1];
}
return retVal;
}
범위는 가장 큰 수 빼기 가장 작은 수로 구했습니다.
int32_t find_range(const std::vector<int32_t>& ElemVec) {
const int32_t maxVal = *max_element(std::begin(ElemVec), std::end(ElemVec));
const int32_t minVal = *min_element(std::begin(ElemVec), std::end(ElemVec));
return (maxVal - minVal);
}
전체 코드는 다음과 같습니다
#include <iostream>
#include <string>
#include <sstream>
#include <vector>
#include <array>
#include <stack>
#include <queue>
#include <map>
#include <algorithm>
#include <numeric>
#include <cmath>
#include <regex>
namespace kimc::lib {
int32_t find_mean(const std::vector<int32_t> &ElemVec) {
int32_t sum = 0;
const size_t vecSize = ElemVec.size();
for (size_t i = 0; i < vecSize; i++)
{
sum += ElemVec[i];
}
const int32_t avg = floor((sum / static_cast<double_t>(vecSize)) + 0.5);
return avg;
}
int32_t find_median(const std::vector<int32_t>& ElemVec) {
const size_t vecSize = ElemVec.size();
int32_t retVal = 0;
if ((vecSize % 2) == 0)
{
retVal = floor(((ElemVec[vecSize / 2 - 1] + ElemVec[vecSize / 2]) / 2) + 0.5);
}
else
{
retVal = ElemVec[vecSize / 2];
}
return retVal;
}
int32_t find_most_frequent_value(const std::vector<int32_t>& ElemVec) {
std::map<int32_t, int32_t> counterMap;
for (auto it : ElemVec)
{
++counterMap[it];
}
int32_t maxVal = 0;
std::vector<int32_t> maxValVec;
for (auto it : counterMap)
{
if (it.second >= maxVal)
{
if (it.second != maxVal)
{
maxVal = it.second;
maxValVec.clear();
}
maxValVec.push_back(it.first);
}
}
int32_t retVal = maxValVec[0];
if (maxValVec.size() >= 2)
{
retVal = maxValVec[1];
}
return retVal;
}
int32_t find_range(const std::vector<int32_t>& ElemVec) {
const int32_t maxVal = *max_element(std::begin(ElemVec), std::end(ElemVec));
const int32_t minVal = *min_element(std::begin(ElemVec), std::end(ElemVec));
return (maxVal - minVal);
}
}
int main()
{
// 입력 최적화
std::cin.tie(NULL);
std::ios::sync_with_stdio(false);
std::string inputStr;
std::getline(std::cin, inputStr);
std::stringstream ss(inputStr);
size_t ElemVecSize;
ss >> ElemVecSize;
ss.seekg(0);
std::vector<int32_t> ElemVec;
for (size_t i = 0U; i < ElemVecSize; i++)
{
std::getline(std::cin, inputStr);
std::stringstream ss(inputStr);
int32_t elemVal;
ss >> elemVal;
ElemVec.push_back(elemVal);
}
// 데이터를 작은 수부터 큰 수 순서대로 정렬
sort(ElemVec.begin(), ElemVec.end());
std::cout << kimc::lib::find_mean(ElemVec) << "\n";
std::cout << kimc::lib::find_median(ElemVec) << "\n";
std::cout << kimc::lib::find_most_frequent_value(ElemVec) << "\n";
std::cout << kimc::lib::find_range(ElemVec) << "\n";
}
읽어주셔서 감사합니다
무엇인가 얻어가셨기를 바라며
오늘도 즐거운 코딩 하시길 바랍니다 ~ :)
참조 및 인용
C++ Primer
Introduction to Algorithms
https://codemasterkimc.tistory.com/35
C++ 이론을 배울수 있는 곳 정리
개요 C++을 배우는 책, 강의, 블로그, 링크 등을 공유합니다. (링크 및 간략한 설명을 하였으나 만약 원작자가 링크를 거는것을 원치 않을 경우 연락주시기 바랍니다.) 서적 https://www.amazon.com/Prime
codemasterkimc.tistory.com
https://codemasterkimc.tistory.com/50
300년차 개발자의 좋은 코드 5계명 (Clean Code)
이번 글을 통해 배워갈 내용 좋은 코드(Clean Code)를 작성하기 위해 개발자로서 생각해볼 5가지 요소를 알아보겠습니다. 개요 좋은 코드란 무엇일까요? 저는 자원이 한정적인 컴퓨터 세상에서 좋
codemasterkimc.tistory.com
'C++ > C++ 알고리즘' 카테고리의 다른 글
백준 11970번 Fence Painting C++ 구현해보기 (0) | 2021.10.31 |
---|---|
백준 5430번 AC C++ 구현해보기 (0) | 2021.10.30 |
백준 11944번 NN C++ 구현해보기 (0) | 2021.10.30 |
백준 1568번 새 C++ 구현해보기 (0) | 2021.10.29 |
백준 5522번 카드게임 C++ 구현해보기 (0) | 2021.10.24 |
```
백준 2108번 통계학 C++ 구현해보기
```
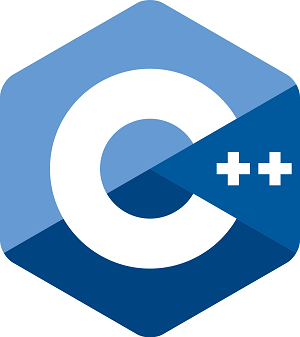
이번 글을 통해 배워갈 내용
- 백준 2108번 풀이
https://www.acmicpc.net/problem/2108
2108번: 통계학
첫째 줄에 수의 개수 N(1 ≤ N ≤ 500,000)이 주어진다. 단, N은 홀수이다. 그 다음 N개의 줄에는 정수들이 주어진다. 입력되는 정수의 절댓값은 4,000을 넘지 않는다.
www.acmicpc.net
백준 2108번 통계학은
난이도 실버 등급의 문제로서
설명
산술평균 Average(Mean),
중앙값 Median
최빈값 most frequent value
범위 range
를 구해주면 되는 문제입니다.
30분 정도 위에 링크를 방문하셔서 풀어보시고
안 풀리시는 경우에만 아래 해답을 봐주시면 감사하겠습니다.
산술평균은 간단하게 N개의 수의 합을 N으로 나눠주면 됩니다.
int32_t find_mean(const std::vector<int32_t> &ElemVec) {
int32_t sum = 0;
const size_t vecSize = ElemVec.size();
for (size_t i = 0; i < vecSize; i++)
{
sum += ElemVec[i];
}
const int32_t avg = floor((sum / static_cast<double_t>(vecSize)) + 0.5);
return avg;
}
중앙값은 데이터를 오름차순으로 정렬 후 데이터의 개수가 홀수면 중앙값, 짝수면 중앙에 있는 두 수의 합 나누기 2를 해주면 됩니다.(정렬된 데이터를 넣는다고 가정하고 함수를 작성했고 입력하는 곳에서 sort를 사용해 정렬했습니다)
int32_t find_median(const std::vector<int32_t>& ElemVec) {
const size_t vecSize = ElemVec.size();
int32_t retVal = 0;
if ((vecSize % 2) == 0)
{
retVal = floor(((ElemVec[vecSize / 2 - 1] + ElemVec[vecSize / 2]) / 2) + 0.5);
}
else
{
retVal = ElemVec[vecSize / 2];
}
return retVal;
}
최빈값은 가장 많이 나타나는 숫자를 구해주면 되는데 해당 문제의 경우 2개 이상일 경우 가장 많이 나타나는 수가 2개 이상일 경우 오름차순에서 두 번째 수를 반환해주면 됩니다.
int32_t find_most_frequent_value(const std::vector<int32_t>& ElemVec) {
std::map<int32_t, int32_t> counterMap;
for (auto it : ElemVec)
{
++counterMap[it];
}
int32_t maxVal = 0;
std::vector<int32_t> maxValVec;
for (auto it : counterMap)
{
if (it.second >= maxVal)
{
if (it.second != maxVal)
{
maxVal = it.second;
maxValVec.clear();
}
maxValVec.push_back(it.first);
}
}
int32_t retVal = maxValVec[0];
if (maxValVec.size() >= 2)
{
retVal = maxValVec[1];
}
return retVal;
}
범위는 가장 큰 수 빼기 가장 작은 수로 구했습니다.
int32_t find_range(const std::vector<int32_t>& ElemVec) {
const int32_t maxVal = *max_element(std::begin(ElemVec), std::end(ElemVec));
const int32_t minVal = *min_element(std::begin(ElemVec), std::end(ElemVec));
return (maxVal - minVal);
}
전체 코드는 다음과 같습니다
#include <iostream>
#include <string>
#include <sstream>
#include <vector>
#include <array>
#include <stack>
#include <queue>
#include <map>
#include <algorithm>
#include <numeric>
#include <cmath>
#include <regex>
namespace kimc::lib {
int32_t find_mean(const std::vector<int32_t> &ElemVec) {
int32_t sum = 0;
const size_t vecSize = ElemVec.size();
for (size_t i = 0; i < vecSize; i++)
{
sum += ElemVec[i];
}
const int32_t avg = floor((sum / static_cast<double_t>(vecSize)) + 0.5);
return avg;
}
int32_t find_median(const std::vector<int32_t>& ElemVec) {
const size_t vecSize = ElemVec.size();
int32_t retVal = 0;
if ((vecSize % 2) == 0)
{
retVal = floor(((ElemVec[vecSize / 2 - 1] + ElemVec[vecSize / 2]) / 2) + 0.5);
}
else
{
retVal = ElemVec[vecSize / 2];
}
return retVal;
}
int32_t find_most_frequent_value(const std::vector<int32_t>& ElemVec) {
std::map<int32_t, int32_t> counterMap;
for (auto it : ElemVec)
{
++counterMap[it];
}
int32_t maxVal = 0;
std::vector<int32_t> maxValVec;
for (auto it : counterMap)
{
if (it.second >= maxVal)
{
if (it.second != maxVal)
{
maxVal = it.second;
maxValVec.clear();
}
maxValVec.push_back(it.first);
}
}
int32_t retVal = maxValVec[0];
if (maxValVec.size() >= 2)
{
retVal = maxValVec[1];
}
return retVal;
}
int32_t find_range(const std::vector<int32_t>& ElemVec) {
const int32_t maxVal = *max_element(std::begin(ElemVec), std::end(ElemVec));
const int32_t minVal = *min_element(std::begin(ElemVec), std::end(ElemVec));
return (maxVal - minVal);
}
}
int main()
{
// 입력 최적화
std::cin.tie(NULL);
std::ios::sync_with_stdio(false);
std::string inputStr;
std::getline(std::cin, inputStr);
std::stringstream ss(inputStr);
size_t ElemVecSize;
ss >> ElemVecSize;
ss.seekg(0);
std::vector<int32_t> ElemVec;
for (size_t i = 0U; i < ElemVecSize; i++)
{
std::getline(std::cin, inputStr);
std::stringstream ss(inputStr);
int32_t elemVal;
ss >> elemVal;
ElemVec.push_back(elemVal);
}
// 데이터를 작은 수부터 큰 수 순서대로 정렬
sort(ElemVec.begin(), ElemVec.end());
std::cout << kimc::lib::find_mean(ElemVec) << "\n";
std::cout << kimc::lib::find_median(ElemVec) << "\n";
std::cout << kimc::lib::find_most_frequent_value(ElemVec) << "\n";
std::cout << kimc::lib::find_range(ElemVec) << "\n";
}
읽어주셔서 감사합니다
무엇인가 얻어가셨기를 바라며
오늘도 즐거운 코딩 하시길 바랍니다 ~ :)
참조 및 인용
C++ Primer
Introduction to Algorithms
https://codemasterkimc.tistory.com/35
C++ 이론을 배울수 있는 곳 정리
개요 C++을 배우는 책, 강의, 블로그, 링크 등을 공유합니다. (링크 및 간략한 설명을 하였으나 만약 원작자가 링크를 거는것을 원치 않을 경우 연락주시기 바랍니다.) 서적 https://www.amazon.com/Prime
codemasterkimc.tistory.com
https://codemasterkimc.tistory.com/50
300년차 개발자의 좋은 코드 5계명 (Clean Code)
이번 글을 통해 배워갈 내용 좋은 코드(Clean Code)를 작성하기 위해 개발자로서 생각해볼 5가지 요소를 알아보겠습니다. 개요 좋은 코드란 무엇일까요? 저는 자원이 한정적인 컴퓨터 세상에서 좋
codemasterkimc.tistory.com
'C++ > C++ 알고리즘' 카테고리의 다른 글
백준 11970번 Fence Painting C++ 구현해보기 (0) | 2021.10.31 |
---|---|
백준 5430번 AC C++ 구현해보기 (0) | 2021.10.30 |
백준 11944번 NN C++ 구현해보기 (0) | 2021.10.30 |
백준 1568번 새 C++ 구현해보기 (0) | 2021.10.29 |
백준 5522번 카드게임 C++ 구현해보기 (0) | 2021.10.24 |